Configuration¶
mSelect accept an object of options which provides several functionality.
Important
The only option that is required by default is the url
, which populate the mSelect.
Options¶
below is simple example that contains all options available. After the example, we explain the role of some options with examples.
var country_id = $('#country_id').mSelect({
table_id: 'dt_country', // The id for the datatable (optional)
columns: ['Name'], // The label of the columns, (optional: if you have only a Name column)
url: 'ajax/countries.php', // The ajax url (required)
data: {}, // The data to pass via ajax
ajax_var_name: 'ids', // The name of the variable that we pass via ajax url for the selected rows
prependData: null, // Prepend some data to the returned datatable rows
selectedIds: [], // The selected ids to select / return
returnSelectedLabels:{ // You may need to return the selected labels as well
enable: false,
indexLabel: 1, // The index of the label to return, usually the Name (column 1)
uniqueLabel: false // If true the uniqueSelectedLabels will be returned
},
loadWhenOpen: false, // If true: the mSelect will be populated only when we open it
disableIfEmpty: false, // Disable the mSelect if no data
disable: false, // Disable the mSelect
reset: false, // Reset the mSelect
minWidth: 350, // The min Width for mSelect dropdown container
buttonWidth: null, // The buttonWidth for the multislect (possible values: null, 100%)
cssNotEmpty: null, // Apply a style for the mSelect Button when there is selected data
nonSelectedText: null, // The label for the dropdown, by default is: "None selected" if "en" is selected
selectType: 'multi', // Multi select or Single Select (possible values: multi, single)
topNav: false, // Show or not the navigation buttons on the top
btnRefresh: true, // Show or not the Refresh button
btnSelected:{ // Show or not the Selected button
enable: true,
icon1: 'fa fa-eye', // The icon class for show selected button
icon2: 'fa fa-eye-slash', // The icon class for show all button
color_icon1: '#666', // The color for show selected button
color_icon2: '#666' // The color for show selected button
},
applyStyleToDom: { // Apply a css style to an element in the DOM (class or id) when the mSelect is opened, and remove the style when the mSelect is closed.
item: null,
style: null
},
lengthMenu: [10, 20, 50], // Datatable lenght page
order: [[1, 'asc']], // Datatable order column
lang: 'en', // Language of the plugin: en, fr, es, pt, ru, zh, ja
onDropdownShow: function() { // callback functions
// do something here..
},
onDropdownHide: function(selectedIds, selectedLabels, uniqueSelectedLabels) {
// do something here..
},
onChange : function(selectedIds, selectedLabels, uniqueSelectedLabels) {
// do something here..
}
});
table_id¶
- The id for the datatable (optional),
- By default a unique id will be generated automaticaly by mSelect.
- But you always can specify your custom id if you want.
columns¶
- The label of the columns, (optional: if you have only a Name column)
- You always can custom the column Name or add more columns.
$('#state_id').mSelect({
columns: ['Sate Name', 'Country Name'],
url: 'ajax/states.php'
});
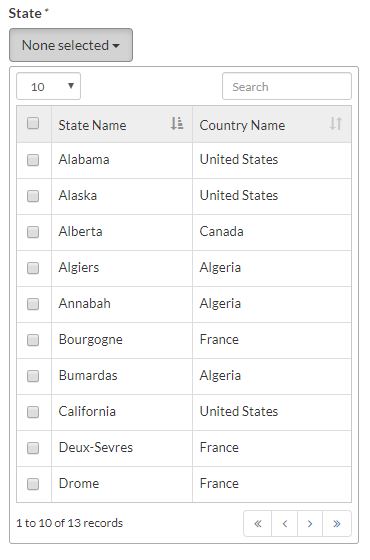
data¶
The data is an object that we may need to pass via ajax to the server side script.
$('#state_id').mSelect({
url: 'ajax/states.php',
data: {
var1: "value1",
var2: "value2",
}
});
In your PHP Server Side Code, You may have something like this:
<?php
$var1 = isset($_GET['var1']) && !empty($_GET['var1']) ? $_GET['var1'] : null;
$var2 = isset($_GET['var2']) && !empty($_GET['var2']) ? $_GET['var2'] : null;
ajax_var_name¶
The name of the variable that we pass via ajax url to see the the selected rows when we click on the Show selected button.
By default the name of this var is: ids
This option work with the options: selectedIds and btnSelected.
Example:
We gonna load the Select for the list of the countries, and let’s suppose that we need some countries to be selected when the mSelect load, and when we click to Show selected button, this viariable is fired
$('#state_id').mSelect({
url: 'ajax/states.php',
ajax_var_name: 'ids', //
selectedIds: ["3","8","44","57"], // The ids of the countries that needs to be selected by default
btnSelected: { // The Button Selected must be enabled if you want to use ajax_var_name
enable: false
},
});
In your PHP Server Side Code, You may have something like this:
<?php
//.....
$selectedIds = isset($_GET['ids']) && !empty($_GET['ids']) ? $_GET['ids'] : [];
$whereResult = !empty($selectedIds) ? "id IN($selectedIds)" : null;
echo json_encode(
Datatables::complex($_GET, $sql_details, $table, $primaryKey, $columns, $whereResult, $whereAll = null);
);
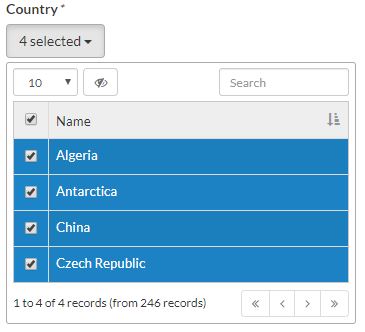
Warning
Please don’t confuse this variable with the data. this variable should not be passed via data object.
prependData¶
Sometimes You may need to prepend some data to the returned datatable rows.
Example:
Let say we need to prepend a value: Undefined
$('#state_id').mSelect({
url: 'ajax/countries.php',
prependData: {'null': 'Undefined'},
});
In your PHP Server Side Code, You may have something like this:
<?php
//.....
//.....
$response = Datatables::complex($_GET, $sql_details, $table, $primaryKey, $columns, $whereResult = null, $whereAll = null);
if(isset($_GET['prependData'])){
foreach ($_GET['prependData'] as $key => $value) {
array_unshift($response['data'], [$key, $value]);
}
}
//.....
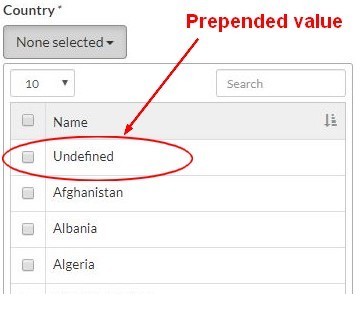
selectedIds¶
Conatains the selected ids that need to be selected and the ids that need to be returned of we need to select more.
This option is related to the Events onDropdownHide and onChange.
returnSelectedLabels¶
By default the selected ids is returned, but sometimes we may need to return the selected labels as well,
returnSelectedLabels:{
enable: true,
indexLabel: 1,
uniqueLabel: true
},
- The
indexLabel
: (by default = 1) the index of the column Name the we need to return. - The
uniqueLabel
: (by default = false) turn this option totrue
to make sure you get the unique Labels as well.
This option is related to the Events onDropdownHide and onChange.
loadWhenOpen¶
- By default =
false
- Turn this option to
true
if you want to populate the mSelect only when you open it.
disableIfEmpty¶
- By default =
false
- Disable the mSelect if there is no data
disable¶
- By default =
false
- Disable the mSelect
reset¶
- By default =
false
- Reset the mSelect
This option may be useful when you reload the mSelect using ajax.
minWidth¶
- By default = 350px
- The min Width for mSelect dropdown container
Example:
$('#state_id').mSelect({
url: 'ajax/countries.php',
minWidth: 800 // the width of the mSelect container is 800px
});
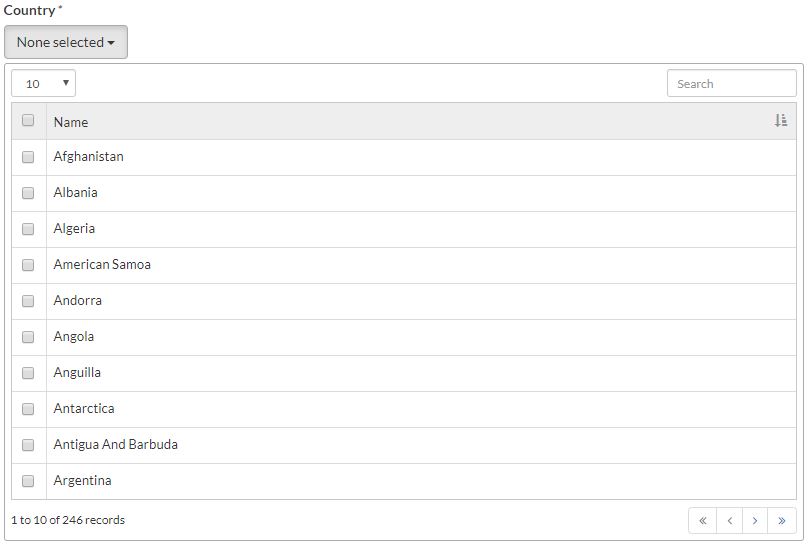
buttonWidth¶
The buttonWidth for the multislect (possible values: null
, 100%
)
Example:
$('#state_id').mSelect({
url: 'ajax/countries.php',
buttonWidth: '100%'
});
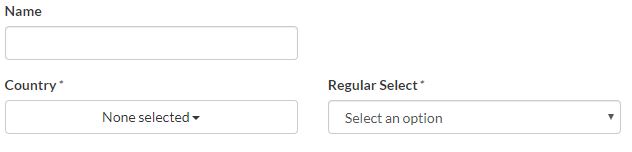
cssNotEmpty¶
Apply a style for the mSelect Button when there is selected data
Example:
$('#state_id').mSelect({
url: 'ajax/countries.php',
cssNotEmpty: 'background:yellow;border:1px solid blue',
});
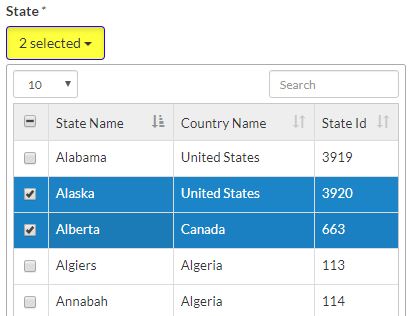
nonSelectedText¶
The label for the dropdown, by default is: “None selected” if “en” is selected
selectType¶
Multi select or Single Select (possible values: multi
, single
)
btnRefresh¶
- By default =
false
- Show or not the Refresh button
Example:
$('#state_id').mSelect({
url: 'ajax/countries.php',
btnRefresh: true, //show the button refresh
});
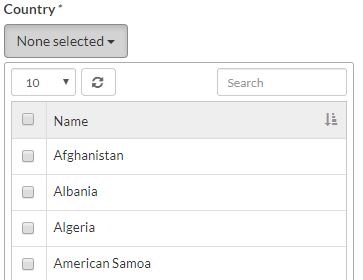
btnSelected¶
This option is an like an object
btnSelected:{ // Show or not the Selected button
enable: true,
icon1: 'fa fa-eye', // The icon class for show selected button
icon2: 'fa fa-eye-slash', // The icon class for show all button
color_icon1: '#666', // The color for show selected button
color_icon2: '#666' // The color for show selected button
},
Example 1:
$('#state_id').mSelect({
url: 'ajax/countries.php',
btnSelected: {// Show the Selected button
enable: true,
},
});
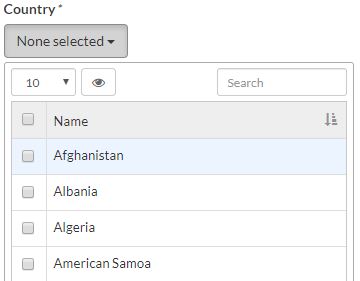
Example 2:
$('#state_id').mSelect({
url: 'ajax/countries.php',
btnSelected: {// Show the Selected button
enable: true,
icon1: 'fa fa-filter',
icon2: 'fa fa-times',
color_icon1: 'blue',
color_icon2: 'red',
},
});
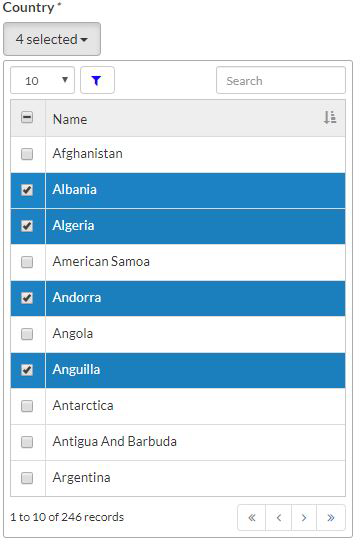
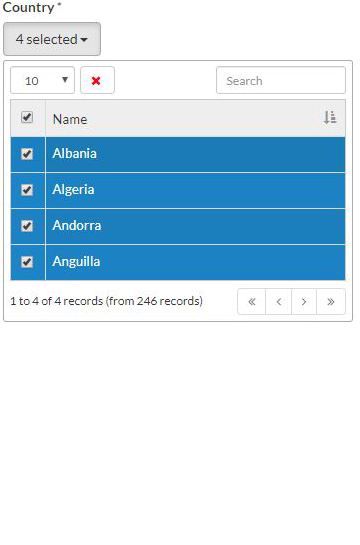
applyStyleToDom¶
Apply a css style to an element in the DOM (class or id) when the mSelect is opened, and remove the style when the mSelect is closed.
Example 1:
$('#country_id').mSelect({
url: 'ajax/countries.php',
applyStyleToDom: {
item: '.mydiv',
style: 'background: yellow;'
},
});
In the example above when the user open the mSelect, a backgroud yellow will be applied automatically to the div with the class .mydiv, and when the user close the mSelect the background will be removed.
Example 2:
$('#location_id').mSelect({
url: 'ajax/process.php',
applyStyleToDom: {
item: '.content-wrapper',
style: 'overflow:auto;'
},
});
order¶
The Datatable order column
lang¶
- Supported Languages:
en
(English)fr
(French)es
(Spanish)pt
(Portuguese)ru
(Russian)zh
(Chinese)ja
(Japanese)
Example:
$('#state_id').mSelect({
url: 'ajax/countries.php',
lang: 'fr'
});
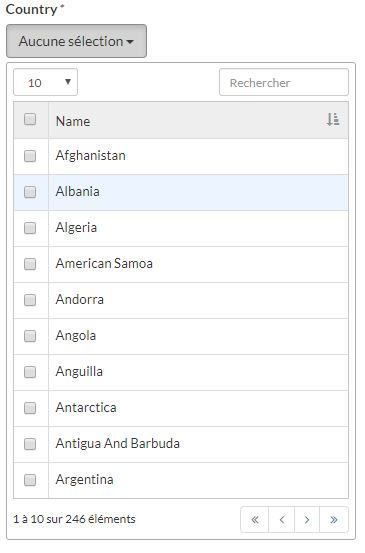