Methods¶
getSelectedIds¶
$("button").click(function(){
var ids = country_id.getSelectedIds(); // call the getSelectedIds method
console.log(ids);
});
getSelectedLabels¶
$("button").click(function(){
var labels = country_id.getSelectedLabels(); // call the getSelectedLabels method
console.log(labels);
});
getUniqueSelectedLabels¶
$("button").click(function(){
var labels = country_id.getUniqueSelectedLabels(); // call the getUniqueSelectedLabels method
console.log(labels);
});
getSelectInfos¶
get all the infos from the methods above.
$("button").click(function(){
var infos = country_id.getSelectInfos(); // call the getSelectInfos method
console.log(infos);
});
setSelectedIds¶
This method accept an optional parameter in order to select the corresponding labels.
element.setSelectedIds(array_of_ids, array_of_labels);
$("button").click(function(){
country_id.setSelectedIds(["231", "30"]); // call the setSelectedIds method to select the ids 231 and 30
});
$("button").click(function(){
country_id.setSelectedIds(["231", "30"], ["United States", "Brazil"]); // call the setSelectedIds method to select the ids 231, 30 with the corresponding labels: United States, Brazil
});
You can do something like that with this method:
$("button").click(function(){
country_id.setSelectedIds(null); // equivalent to mSelect('reset');
});
Or:
$("button").click(function(){
country_id.setSelectedIds([]); // equivalent to mSelect('reset');
});
Warning
If selectType: 'single'
setSelectedIds method will select only the first element
setLanguage¶
- The Language is usually loaded when you load the plugin for first time, but you always can change it if needed.
- The supported Languages are:
en
(English)fr
(French)es
(Spanish)pt
(Portuguese)ru
(Russian)zh
(Chinese)ja
(Japanese)
$("button").click(function(){
country_id.setLanguage('es'); // call the setLanguage method to change the default Language to Spanish
});
setCSS¶
$("button#add-css").click(function(){
country_id.setCSS('background:yellow'); // call the setCSS method to apply a style to the mSelect button
});
$("button#remove-css").click(function(){
country_id.setCSS(null); // call the setCSS method to remove the style from the mSelect button
});
mSelect¶
reset¶
$("button").click(function(){
state_id.mSelect('reset'); // reset the mSelect
});
enable¶
$("button").click(function(){
state_id.mSelect('enable'); // enable the mSelect
});
disable¶
$("button").click(function(){
state_id.mSelect('disable'); // disable the mSelect
});
resable¶
$("button").click(function(){
state_id.mSelect('resable'); // reset and disable the mSelect
});
show¶
$("button").click(function(){
state_id.mSelect('show'); // show the mSelect
});
hide¶
$("button").click(function(){
state_id.mSelect('hide'); // hide the mSelect
});
refresh¶
refresh accept a second parameter which is an object that accept several attributes.
Example 1:
$("button").click(function(){
state_id.mSelect('refresh', {
data: {'country_id': 3}
});
});
Example 2:
$("button").click(function(){
state_id.mSelect('refresh', {
data: {'country_id': 3},
disable: false
});
});
Example 3:
$("button").click(function(){
state_id.mSelect('refresh', {
data: {'country_id': 3},
selectedIds: ["663", "113", "114"],
cssNotEmpty: 'background: yellow',
disable: false,
btnRefresh: false
});
});
Advanced Methods¶
Important
- Every mSelect in the DOM has a unique ID we called
plugin ID
, witch allow you to access to different items inside the mSelect as needed. - All the ids / classes for the items (div, span, select, input, table) inside the mSelect are based on the
plugin ID
.
getPluginId¶
$("button").click(function(){
var plugin_id = country_id.getPluginId(); // this method may be useful for advanced use
console.log(plugin_id);
});
getTableId¶
$("button").click(function(){
var table_id = country_id.getTableId(); // this method may be useful for advanced use
console.log(table_id);
});
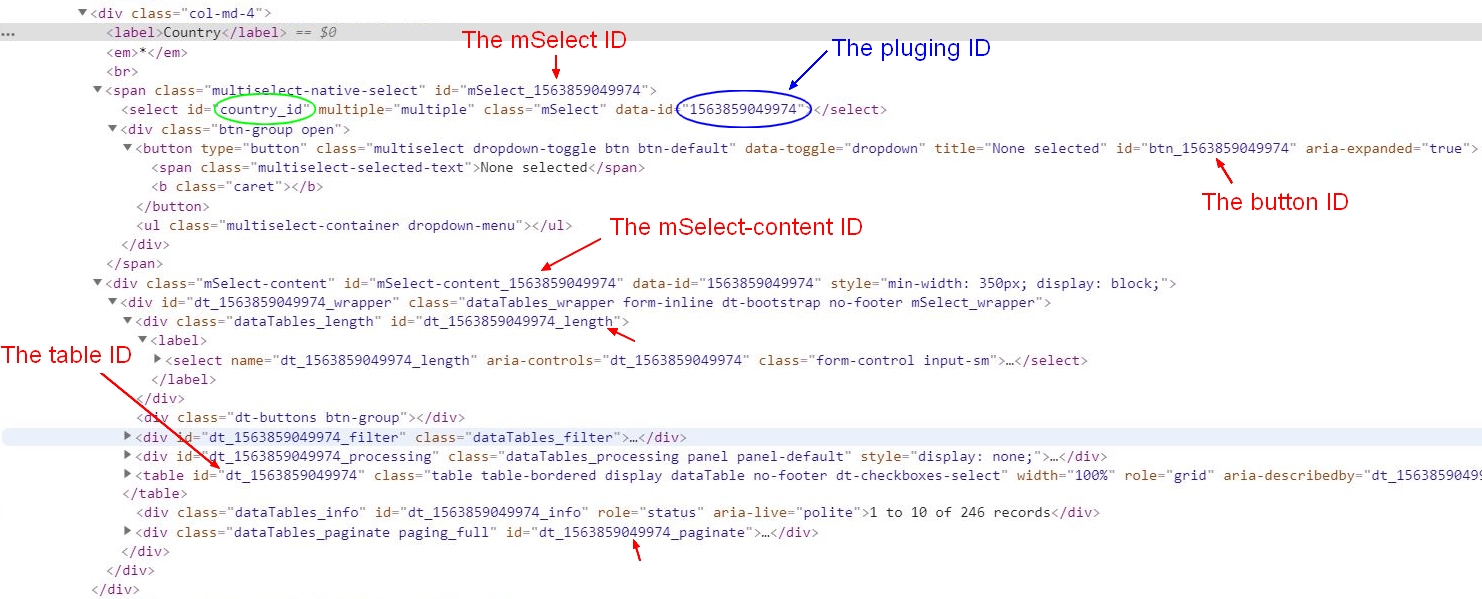